Handling of special assignment types¶
When dealing with different types, you will encounter some special types of operations, such as void * , function pointers, system types and other special types. For these special types, wings provide different operations for special processing.
Function parameter is void * and function pointer¶
For the types of function parameters of void * and function pointers, wings first uses static analysis technology to obtain the specific assignment types when the function parameters are void * and function pointers. For example, the function shown in Figure 21 below:
void func(void *p);
callFunc(int *p);
int callFunc(int *p)
{
char *s ="abc";
func(s);
return 0;
}
int func(int(*f)(int));
void functest();
void functest()
{
func(fun);
}
Through static analysis, Wings analyzed that the assignment type of func at the called place was char *. During the assignment process, the parameter of func was assigned to char *. Wings mark the specific assignment type of void * on the data table interface.For some types that cannot be determined by analysis technology, wings will display all relevant functions on the interface, the user will select the required type, and the driver will process the corresponding type. As shown in Figure 22 below:
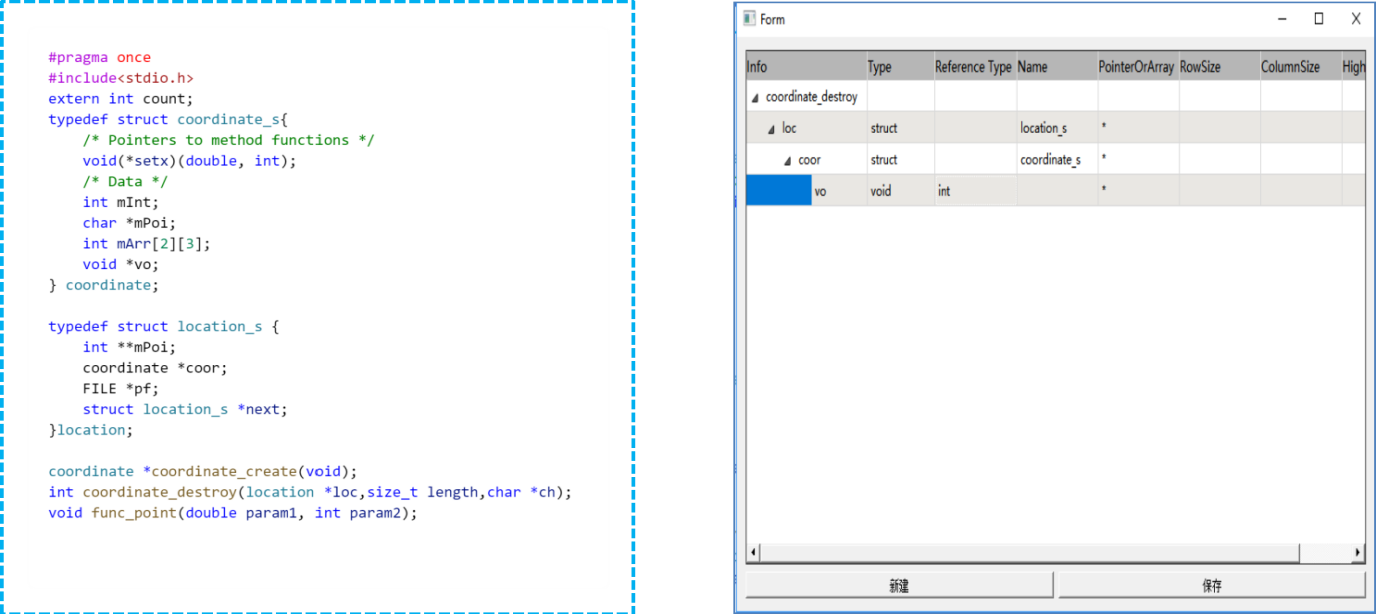
Structure linked list¶
For the linked list type, a more flexible assignment method is adopted. Taking into account some factors in actual application, we have a two-layer structure for default assignment of the linked list type. In the actual testing process, users can automatically add nodes as needed.
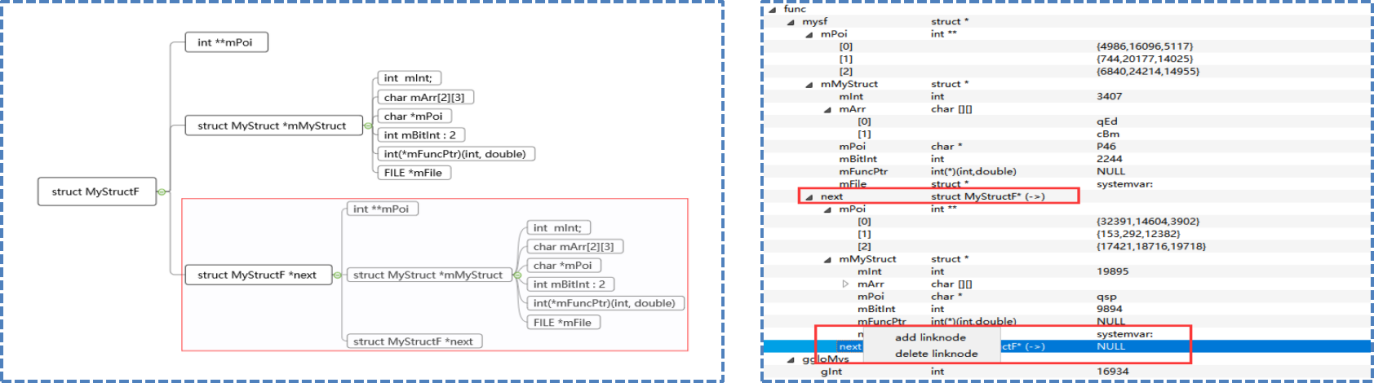
Structures and classes are system variables¶
Wings do special template processing for the header files of some C ++ standard libraries and some third-party libraries. In Table 4 below, some special system variables are defined.
C | C++ |
---|---|
The complex type ( FILE ) used in the C language standard library | STL(std::array、std::forward_list、std::vector Class types in the C ++ standard library ( iostream ) STL ( std :: array , std :: forward_list , std :: vector ) |
Third-party libraries ( cJSON) | Third-party libraries called by C ++ |
Complex types where users need special assignments | Class types where users need special assignments |
- When encountering a special type of assignment, such as sockaddr_in in the following figure, first of all, this type is marked as a type in the system header file and requires special treatment.
- Member variables in sockaddr_in require user-defined assignment
- First, wings detects that sockaddr_in needs special treatment, and it will display this variable in the interface of the template class.
- Then for the variables that need special treatment, such as sin_family, etc., the user needs to configure for the required type.
- After completing configuration, returns the corresponding sockaddr_in structural body to the object.
- Driver code is generated, the function written by the user will be called, and the variables that need to be filled, such as the sin_family value, are automatically generated by us.
Wings are for special templates and have a simple interface for configuration processing.
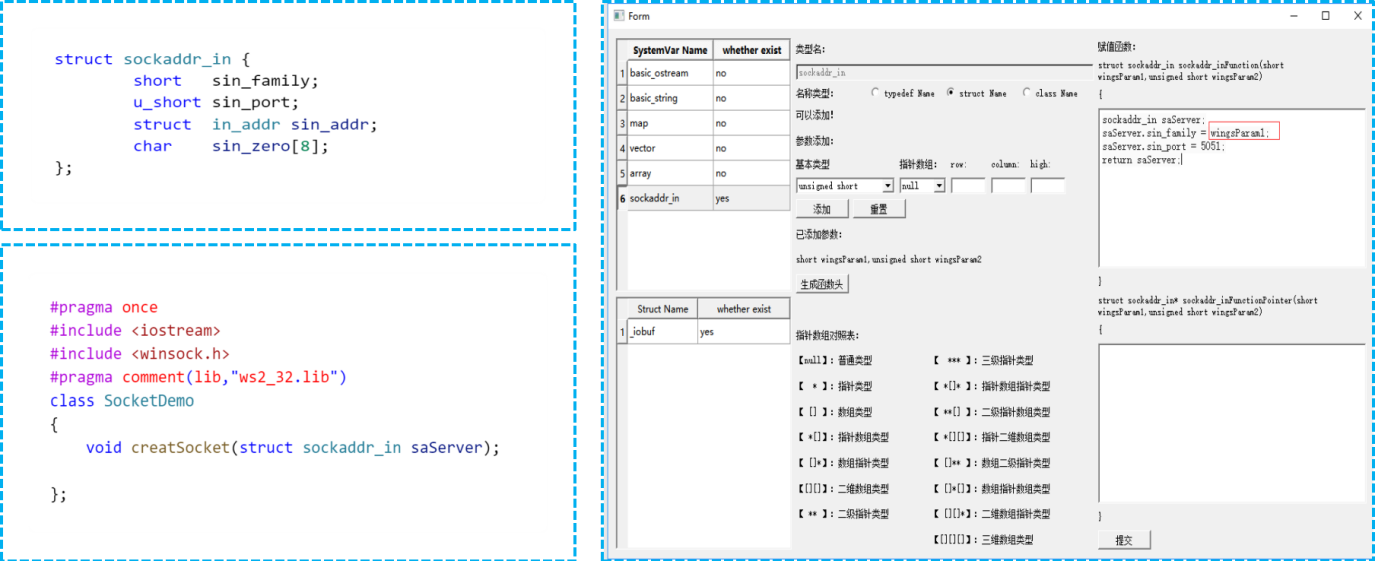
stl standard template library¶
For the standard container in C ++ , we expand and assign the types in the container, and generate a set of values by default. You can click on the interface to add according to your needs.
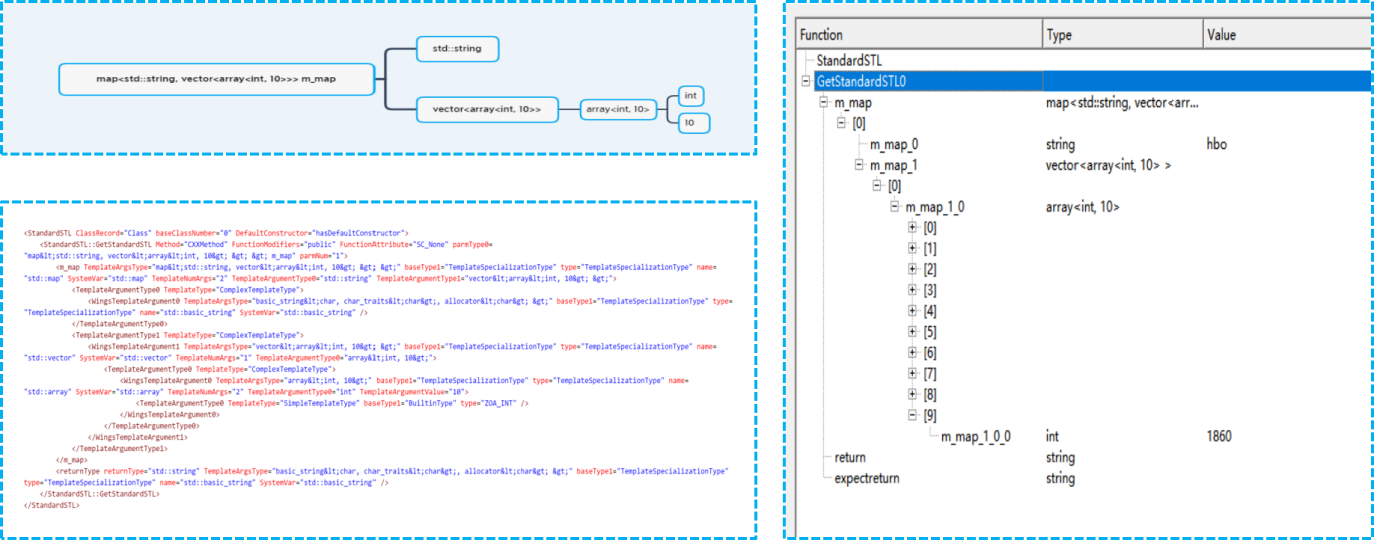
C ++ custom template class¶
For the template type whose parameters are customized, we will analyze the specific type of the template class. For example, the type of the template class in GetTest in Figure 29 is int and double , and the type in GetTestDemo is std :: string and double . Insert a constructor CustomTemplateClass , when actually constructing the template class object, call the specific assignment type to construct.
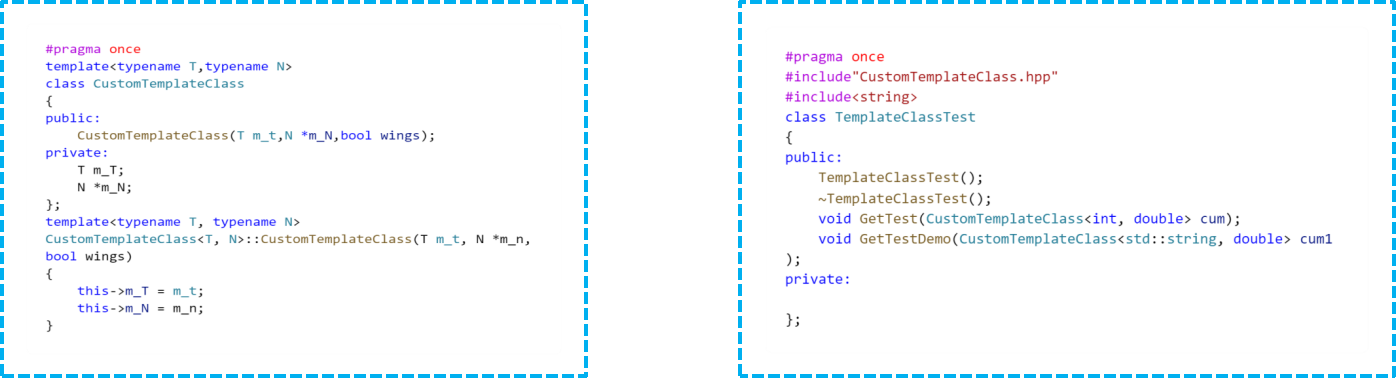